C++20: Basic Chrono Terminology
To get the most out of the chrono extension in C++20, a basic understanding of the chrono terminology is essential.
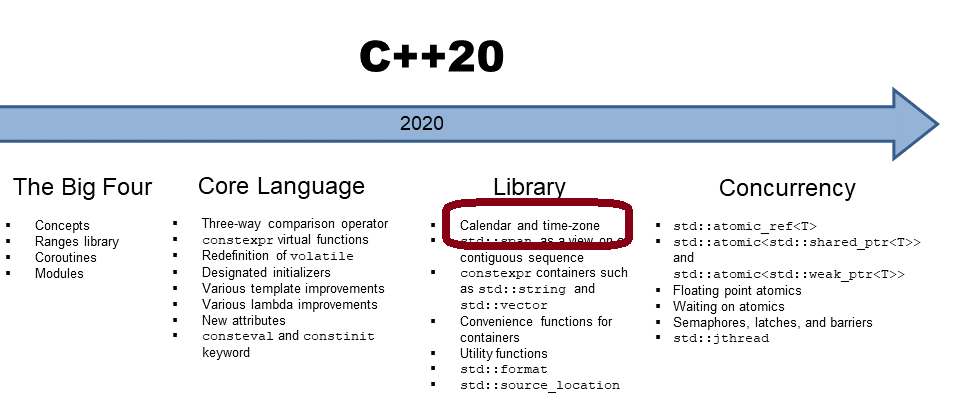
Basic Chrono Terminology
Essentially, the time-zone functionality (C++20) is based on the calendar functionality (C++20) and the calendar functionality (C++20), which are based on the chrono functionality (C++11). Consequently, this basic chrono terminology starts with the three C++11 components time point, time duration, and clock.
- A time point has a starting point, the so-called epoch, and an additional time duration since the epoch.
- A time duration is the difference between two time points. The number of ticks of the clock defines the time duration.
- A clock has a starting point (epoch) and a tick to calculate the current time point.
You can subtract time points and get a time duration. You get a new time point when you add a time duration to a time point. A year is the typical accuracy of the clock and the measure of the time duration.
I will use the three concepts to present the lifetime of the 2011 died father of the programming language C: Dennis Ritchie. For simplicity reasons, I’m only interested in the years.
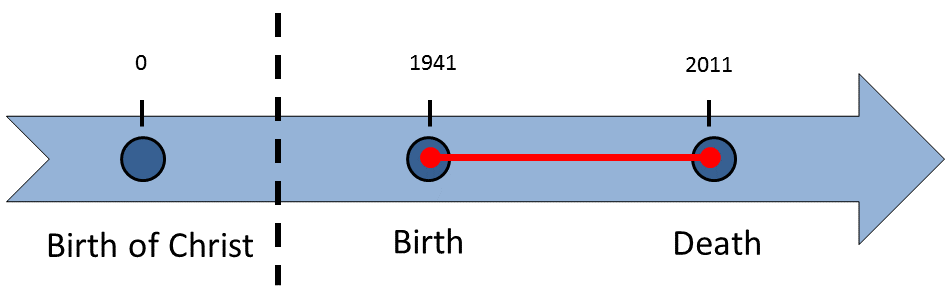
Dennis Ritchie became 70 years old. The birth of Christ is the epoch. Combining the epoch with the time duration gives me the time points 1941 and 2011. Subtraction of the time point 1941 from 2011 provides the time duration in Year’s accuracy.
C++11 has the three clocks std::chrono::system_clock
, std::chrono::steady_clock,
and std::chrono::high_resolution_clock
. The time duration can be positive and negative. Each of the three clocks has a member function now
for returning the current time point.
If you want more details about time point, time duration, and clock, read my previous posts:
C++20 adds new components to the chrono library:
- The time of day is the time duration since midnight, split into hours, minutes, seconds, and fractional seconds.
- Calendar stands for various calendar dates such as year, month, weekday, or the nth day of a week.
- A time zone represents a time specific to a geographic area.
- A zoned time combines a time point with a time zone.
Time is a Mysterium
Honestly, time, for me, is a mystery. On the one hand, each of us has an intuitive idea of time; conversely, defining it formally is exceptionally challenging. For example, the three components, time point, time duration, and clock, depend on each other.
First, let me present the basic types and literals.
Basic Types and Literals
This section includes the basic types and literals of the previous C++ standards for completeness reasons.
Clocks
Besides the wall clock std::chrono::system_clock, the monotonic clock std::chrono::steady_clock, and the most precise clock std::chrono::high_resolution_clock in C++11, C++20 supports five additional clocks. The following table shows the characteristics of all C++ clocks and their epoch.
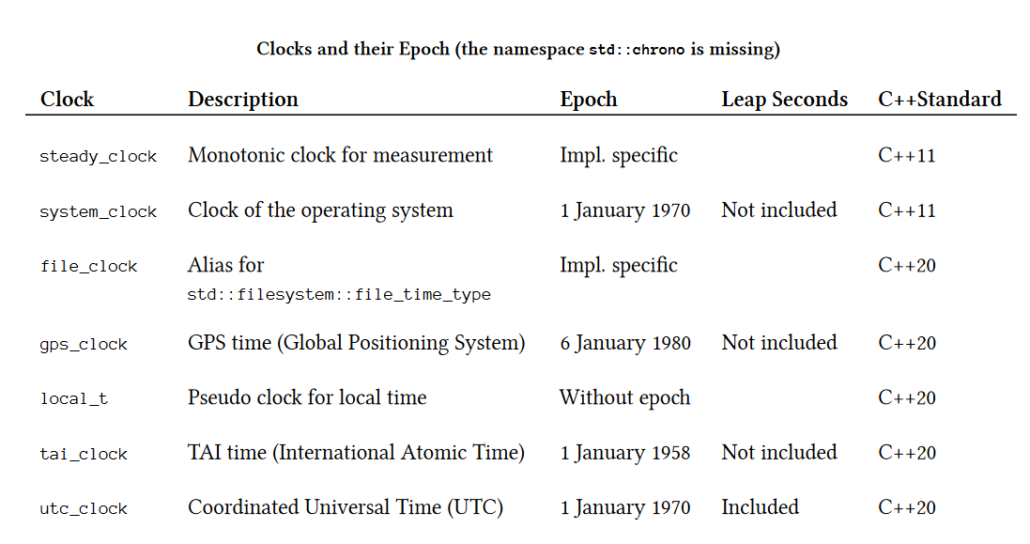
The clocks std::chrono::steady_clock
, and std::chrono::file_clock
have an implementation specified epoch. The epochs of std::chrono::system_clock, std::chrono::gps_clock, std::chrono::tai_clock
, and std::chrono::utc_clock
start at 00:00:00. std::chrono::file_clock
is the clock for file system entries.
Additionally, C++11 supports the std::chrono::high_resolution_clock
. This clock is on all implementations not implemented and is only an alias for std::chrono::steady_clock
or std::chrono::high_resolution_clock.
You can convert a time point between the clocks.
Modernes C++ Mentoring
Do you want to stay informed: Subscribe.
Conversion of time points between clocks
Thanks to the function std::chrono::clock_cast,
you can convert time points between the clocks having an epoch. These are the clocks std::chrono::system_clock, std::chrono::utc_clock, std::chrono::gps_clock
, and std::chrono::tai_clock
. Additionally, std::chrono::file_clock
supports conversion.
The following program converts the time point 2021-8-5 17:00:00
between the various clocks.
// convertClocks.cpp #include <iostream> #include <sstream> #include <chrono> int main() { std::cout << '\n'; using namespace std::literals; std::chrono::utc_time<std::chrono::utc_clock::duration> timePoint; std::istringstream{"2021-8-5 17:00:00"} >> std::chrono::parse("%F %T"s, timePoint); auto timePointUTC = std::chrono::clock_cast<std::chrono::utc_clock>(timePoint); std::cout << "UTC_time: " << std::format("{:%F %X %Z}", timePointUTC) << '\n'; auto timePointSys = std::chrono::clock_cast<std::chrono::system_clock>(timePoint); std::cout << "sys_time: " << std::format("{:%F %X %Z}", timePointSys) << '\n'; auto timePointFile = std::chrono::clock_cast<std::chrono::file_clock>(timePoint); std::cout << "file_time: " << std::format("{:%F %X %Z}", timePointFile) << '\n'; auto timePointGPS = std::chrono::clock_cast<std::chrono::gps_clock>(timePoint); std::cout << "GPS_time: " << std::format("{:%F %X %Z}", timePointGPS) << '\n'; auto timePointTAI = std::chrono::clock_cast<std::chrono::tai_clock>(timePoint); std::cout << "TAI_time: " << std::format("{:%F %X %Z}", timePointTAI) << '\n'; std::cout << '\n'; }
The function std::chrono::parse
parses the chrono object from the stream. In the following lines, std::chrono::clock_cas
t converts the timePoint
into the specified clock. The following line displays the time point, specifying its date (%F
), its local time representation (%X
), and its time zone abbreviation (%Z
). An upcoming post will provide the details about the format string.
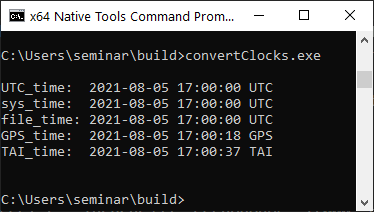
The output may surprise you. GPS time is 18 seconds ahead of the UTC time. TAI time is 37 seconds ahead of the UTC time and 19 seconds ahead of the GPS time.
What’s Next?
I will continue in my next post my journey through the basic types with time durations and time points.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, Philipp Lenk, Charles-Jianye Chen, Keith Jeffery, Matt Godbolt, Honey Sukesan, bruce_lee_wayne, Silviu Ardelean, schnapper79, Seeker, and Sundareswaran Senthilvel.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Modernes C++ GmbH
Modernes C++ Mentoring (English)
Rainer Grimm
Yalovastraße 20
72108 Rottenburg
Mail: schulung@ModernesCpp.de
Mentoring: www.ModernesCpp.org