Time Zones: Online Classes
Today, I will continue to present the functionality of the time zones of the C++20 chrono extension.
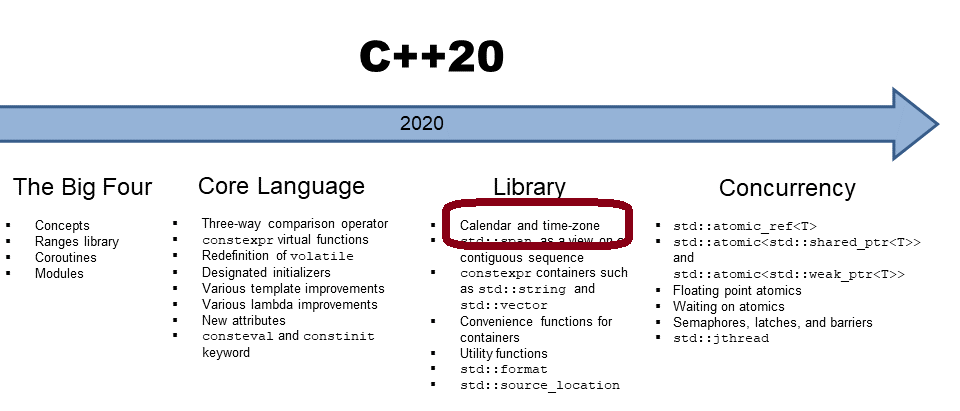
This post is the eight in my detailed journey through the chrono extension in C++20:
- Basic Chrono Terminology
- Basic Chrono Terminology with Time Duration and Time Point
- Time of Day: Details
- Creating Calendar Dates
- Displaying and Checking Calendar Dates
- Query Calendar Dates and Ordinal Dates
- Time Zones: Details
Various Time Zones for Online Classes
The program onlineClass.cpp
answers the following question: How late is it in given time zones when I start an online class at the 7h, 13h,
or 17h
local time (Germany)?
The online class should start on February 1st, 2021, and last 4 hours. Because of daylight savings time, the calendar date is essential to get the correct answer.
#include <chrono> #include <algorithm> #include <iomanip> #include <iostream> using namespace std::chrono_literals; template <typename ZonedTime> auto getMinutes(const ZonedTime& zonedTime) { // (1) return std::chrono::floor<std::chrono::minutes>(zonedTime.get_local_time()); } void printStartEndTimes(const std::chrono::local_days& localDay, // (2) const std::chrono::hours& h, const std::chrono::hours& durationClass, const std::initializer_list<std::string>& timeZones ) { std::chrono::zoned_time startDate{std::chrono::current_zone(), localDay + h}; // (4) std::chrono::zoned_time endDate{std::chrono::current_zone(), localDay + h + durationClass}; std::cout << std::format("Local time: [{}, {}]\n", getMinutes(startDate), getMinutes(endDate)); auto longestStringSize = std::max(timeZones, [](const std::string& a, // (5) const std::string& b) { return a.size() < b.size(); }).size(); std::string formatTimeZones = " {0:<{3}} [{1}, {2}]\n"; // (7) for (auto timeZone: timeZones) { // (6) std::cout << std::vformat(formatTimeZones, std::make_format_args(timeZone, getMinutes(std::chrono::zoned_time(timeZone, startDate)), getMinutes(std::chrono::zoned_time(timeZone, endDate)), longestStringSize + 1)); } } int main() { using namespace std::string_literals; std::cout << '\n'; constexpr auto classDay{std::chrono::year(2021)/2/1}; constexpr auto durationClass = 4h; auto timeZones = {"America/Los_Angeles"s, "America/Denver"s, "America/New_York"s, "Europe/London"s, "Europe/Minsk"s, "Europe/Moscow"s, "Asia/Kolkata"s, "Asia/Novosibirsk"s, "Asia/Singapore"s, "Australia/Perth"s, "Australia/Sydney"s}; for (auto startTime: {7h, 13h, 17h}) { // (3) printStartEndTimes(std::chrono::local_days{classDay}, startTime, durationClass, timeZones); std::cout << '\n'; } std::cout << '\n'; }
Before I dive into the functions getMinutes
(line 1) and printStartEndTimes
(line 2), let me say a few words about the main
function. The main
function defines the day of the class, the duration of the class, and all time zones. Finally, the range-based for-loop (line 3) iterates through all potential starting points for an online class. All necessary information is displayed thanks to the function
(line 2).
The few lines beginning with line (4) calculate the startDate
and endDate
of my training by adding the start and class duration to the calendar date. Both values are displayed with the help of the function getMinutes
(line 1). std::floor<std::chrono::minutes>(zonedTime.get_local_time())
gets the stored time point out of the std::chrono::zoned_time
and truncates the value to the minute resolution. To properly align the output of the program, line (5) determines the size of the longest of all time-zone names. Line (6) iterates through all time zones and displays the name of the time zone and the beginning and end of each online class. A few calendar dates even cross the day boundaries.
The format string in line 7 ” {0:<{3}} [{1}, {2}]\n
” deserves a few words. This lvalue requires the functions std::vformat
and std::make_format_args
. The number in the format string stands for the position in the arguments. The {3}
is a placeholder for the longest time-zone name.
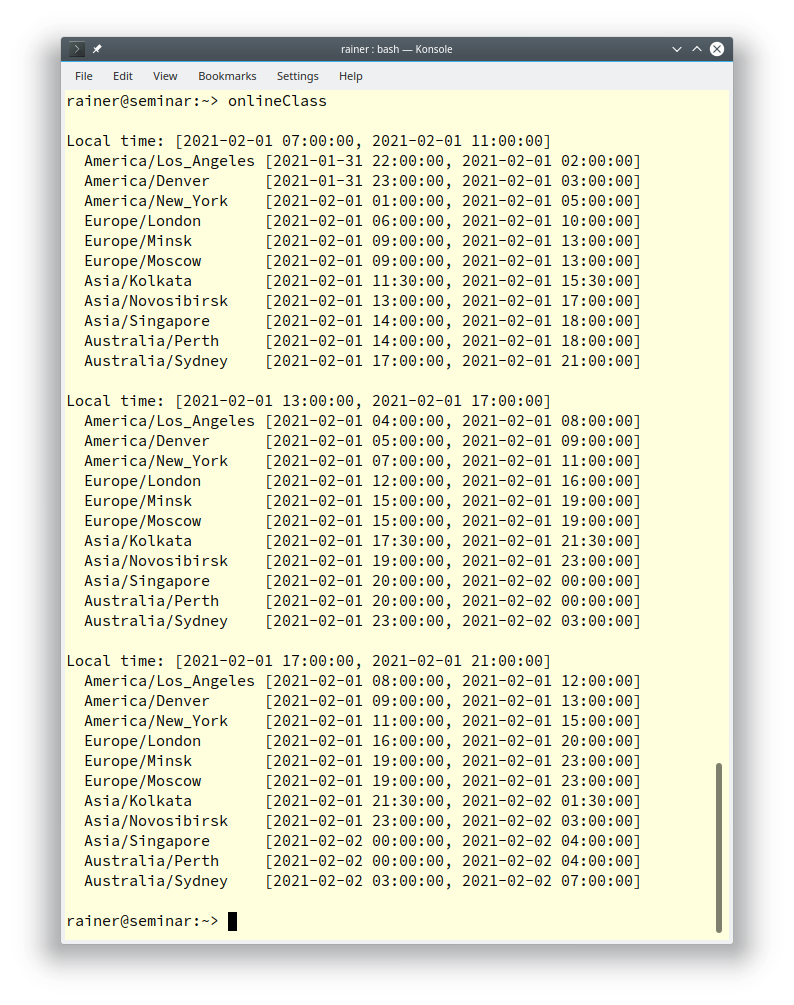
What’s Next?
I/O consists of the reading and writing of the chrono types. The various chrono types support the unformatted writing and the formatted one with the new formatting library. This library also has the function std::chrono::parse()
that makes reading from a stream quite powerful.
Thanks a lot to my Patreon Supporters: Matt Braun, Roman Postanciuc, Tobias Zindl, G Prvulovic, Reinhold Dröge, Abernitzke, Frank Grimm, Sakib, Broeserl, António Pina, Sergey Agafyin, Андрей Бурмистров, Jake, GS, Lawton Shoemake, Jozo Leko, John Breland, Venkat Nandam, Jose Francisco, Douglas Tinkham, Kuchlong Kuchlong, Robert Blanch, Truels Wissneth, Mario Luoni, Friedrich Huber, lennonli, Pramod Tikare Muralidhara, Peter Ware, Daniel Hufschläger, Alessandro Pezzato, Bob Perry, Satish Vangipuram, Andi Ireland, Richard Ohnemus, Michael Dunsky, Leo Goodstadt, John Wiederhirn, Yacob Cohen-Arazi, Florian Tischler, Robin Furness, Michael Young, Holger Detering, Bernd Mühlhaus, Stephen Kelley, Kyle Dean, Tusar Palauri, Juan Dent, George Liao, Daniel Ceperley, Jon T Hess, Stephen Totten, Wolfgang Fütterer, Matthias Grün, Phillip Diekmann, Ben Atakora, Ann Shatoff, Rob North, Bhavith C Achar, Marco Parri Empoli, Philipp Lenk, Charles-Jianye Chen, Keith Jeffery, Matt Godbolt, Honey Sukesan, bruce_lee_wayne, Silviu Ardelean, schnapper79, Seeker, and Sundareswaran Senthilvel.
Thanks, in particular, to Jon Hess, Lakshman, Christian Wittenhorst, Sherhy Pyton, Dendi Suhubdy, Sudhakar Belagurusamy, Richard Sargeant, Rusty Fleming, John Nebel, Mipko, Alicja Kaminska, Slavko Radman, and David Poole.
My special thanks to Embarcadero | ![]() |
My special thanks to PVS-Studio | ![]() |
My special thanks to Tipi.build | ![]() |
My special thanks to Take Up Code | ![]() |
My special thanks to SHAVEDYAKS | ![]() |
Modernes C++ GmbH
Modernes C++ Mentoring (English)
Rainer Grimm
Yalovastraße 20
72108 Rottenburg
Mail: schulung@ModernesCpp.de
Mentoring: www.ModernesCpp.org
Modernes C++ Mentoring
Do you want to stay informed: Subscribe.